Inline adaptive banner ads are types of responsive ads that adjust their size with different device screen widths and display within the Flutter app layout. It seems to be a very interactive user experience while scrolling the app. As the same as a standard adaptive banner ads, it is easy to integrate in your Flutter project.
Table of Contents
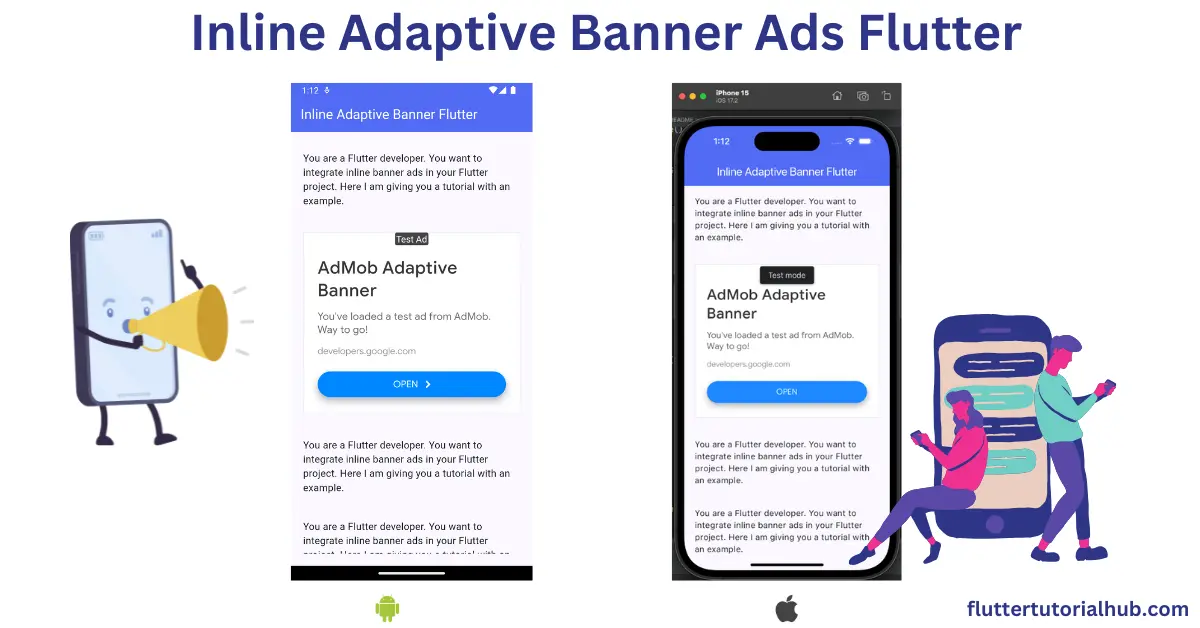
In this article, we will see how to implement inline adaptive banner ads in your Flutter project. We will see a step-by-step code implementation tutorial with examples. Before we dive into code implementation, we well see some benefits for using these ads.
Benefits of using Inline Adaptive Banner Ads in Flutter
There are several benefits of using inline banner ads in your Flutter project. We will see below:
- Responsive Design: It adjusts the width with different devices. It is making more suitable ad units for publishers.
- Better User Experience: While scrolling the content, this kind of ad displays as per spaces between the content. It allows a better user experience with less disturbing.
- High Ad Performance: It displays with fit to app layout. It performs very well, achieving higher performance and click-through rates.
How do I implement inline banner ads in Flutter?
Now we dive into some coding implementation. Follow the below steps:
Step 1: Open and modify the pubspec.yaml file
Let’s start with pubspec.yaml file. Here, we will define the Google mobile ad package and retrieve it in our project. Define the google_mobile_ads package under the dependencies section.
dependencies:
google_mobile_ads: 5.1.0
Step 2: Import header file in main.dart file
import 'dart:developer';
import 'package:flutter/material.dart';
import 'package:google_mobile_ads/google_mobile_ads.dart';
import 'dart:io';
Step 3: Initialize Google Mobile Ads SDK in main function
void main() {
WidgetsFlutterBinding.ensureInitialized();
MobileAds.instance.initialize();
runApp(const InlineAdaptiveBannerApp());
}
Step 4: Initialize the _inlineAdaptiveBannerAd object of the BannerAd class
BannerAd? _inlineAdaptiveBannerAd;
Step 5: Load and Get Inline Banner Ad
void _loadInlineAdaptiveBannerAd() async {
await _inlineAdaptiveBannerAd?.dispose();
setState(() {
_inlineAdaptiveBannerAd = null;
_isLoadedBannerAd = false;
});
AdSize size = AdSize.getCurrentOrientationInlineAdaptiveBannerAdSize(
_adWidthBannerAd.truncate());
_inlineAdaptiveBannerAd = BannerAd(
// TODO: replace this test ad unit with your own ad unit.
adUnitId: 'ca-app-pub-3940256099942544/9214589741',
size: size,
request: const AdRequest(),
listener: BannerAdListener(
onAdLoaded: (Ad ad) async {
log('Inline adaptive banner loaded: ${ad.responseInfo}');
BannerAd bannerAd = (ad as BannerAd);
final AdSize? size = await bannerAd.getPlatformAdSize();
if (size == null) {
log('Error: getPlatformAdSize() returned null for $bannerAd');
return;
}
setState(() {
_inlineAdaptiveBannerAd = bannerAd;
_isLoadedBannerAd = true;
_inlineAdaptiveBannerAdSize = size;
});
},
onAdFailedToLoad: (Ad ad, LoadAdError error) {
log('Inline adaptive banner failedToLoad: $error');
ad.dispose();
},
),
);
await _inlineAdaptiveBannerAd!.load();
}
// Display Ads by calling this widget
Widget _getInlineBannerAdWidget() {
return OrientationBuilder(
builder: (context, orientation) {
if (_currentOrientationBannerAd == orientation &&
_inlineAdaptiveBannerAd != null &&
_isLoadedBannerAd &&
_inlineAdaptiveBannerAdSize != null) {
return Align(
child: SizedBox(
width: _adWidthBannerAd,
height: _inlineAdaptiveBannerAdSize!.height.toDouble(),
child: AdWidget(
ad: _inlineAdaptiveBannerAd!,
),
));
}
// Reload the ad if the orientation changes.
if (_currentOrientationBannerAd != orientation) {
_currentOrientationBannerAd = orientation;
_loadInlineAdaptiveBannerAd();
}
return Container();
},
);
}
Step 6: Modify AndroidManifest.xml
Add test AdMob AppId to your in Android Manifest file by adding the <meta-data> tag inside the <application>
tag.
<manifest>
<application>
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-3940256099942544~3347511713" />
</application>
</manifest>
Step 7: Modify Modify info.plist
Add Admob App ID in the info.plist file.
<key>GADApplicationIdentifier</key>
<string>ca-app-pub-3940256099942544~1458002511</string>
Step 8: Inline Adaptive Banner Ads in Flutter With Example (Full Code)
import 'dart:developer';
import 'package:flutter/material.dart';
import 'package:google_mobile_ads/google_mobile_ads.dart';
import 'dart:io';
void main() {
WidgetsFlutterBinding.ensureInitialized();
MobileAds.instance.initialize();
runApp(const InlineAdaptiveBannerApp());
}
class InlineAdaptiveBannerApp extends StatelessWidget {
const InlineAdaptiveBannerApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Inline Banner Ads',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.indigoAccent),
useMaterial3: true,
),
home: const InlineAdaptiveBannerAppPage(title: 'Inline Adaptive Banner Flutter'),
);
}
}
class InlineAdaptiveBannerAppPage extends StatefulWidget {
const InlineAdaptiveBannerAppPage({super.key, required this.title});
final String title;
@override
State<InlineAdaptiveBannerAppPage> createState() => InlineAdaptiveBannerAppPageState();
}
class InlineAdaptiveBannerAppPageState extends State<InlineAdaptiveBannerAppPage> {
static const _insetsBannerAdHorizontal = 20.0;
static const _insetsBannerAdVertical = 20.0;
BannerAd? _inlineAdaptiveBannerAd;
bool _isLoadedBannerAd = false;
AdSize? _inlineAdaptiveBannerAdSize;
late Orientation _currentOrientationBannerAd;
double get _adWidthBannerAd =>
MediaQuery.of(context).size.width - (2 * _insetsBannerAdHorizontal);
@override
void didChangeDependencies() {
super.didChangeDependencies();
_currentOrientationBannerAd = MediaQuery.of(context).orientation;
_loadInlineAdaptiveBannerAd();
}
// Copy and paste _loadInlineAdaptiveBannerAd() function from Step 5
// Copy and paste _getInlineBannerAdWidget() function from Step 5
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.indigoAccent,
centerTitle: Platform.isAndroid ? false : true,
foregroundColor: Colors.white,
title: Text(widget.title),
),
body: Center(
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: _insetsBannerAdHorizontal,vertical: _insetsBannerAdVertical),
child: ListView.separated(
itemCount: 20,
separatorBuilder: (BuildContext context, int index) {
return Container(
height: 40,
);
},
itemBuilder: (BuildContext context, int index) {
if (index == 10) {
return _getInlineBannerAdWidget();
}
return const Text(
'You are a Flutter developer. You want to integrate inline banner ads in your Flutter project. Here I am giving you a tutorial with an example.',
style: TextStyle(fontSize: 16),
);
},
),
),
));
}
@override
void dispose() {
super.dispose();
_inlineAdaptiveBannerAd?.dispose();
}
}
Step 9: Run the project!
Output for Android device and iPhone 15 simulator
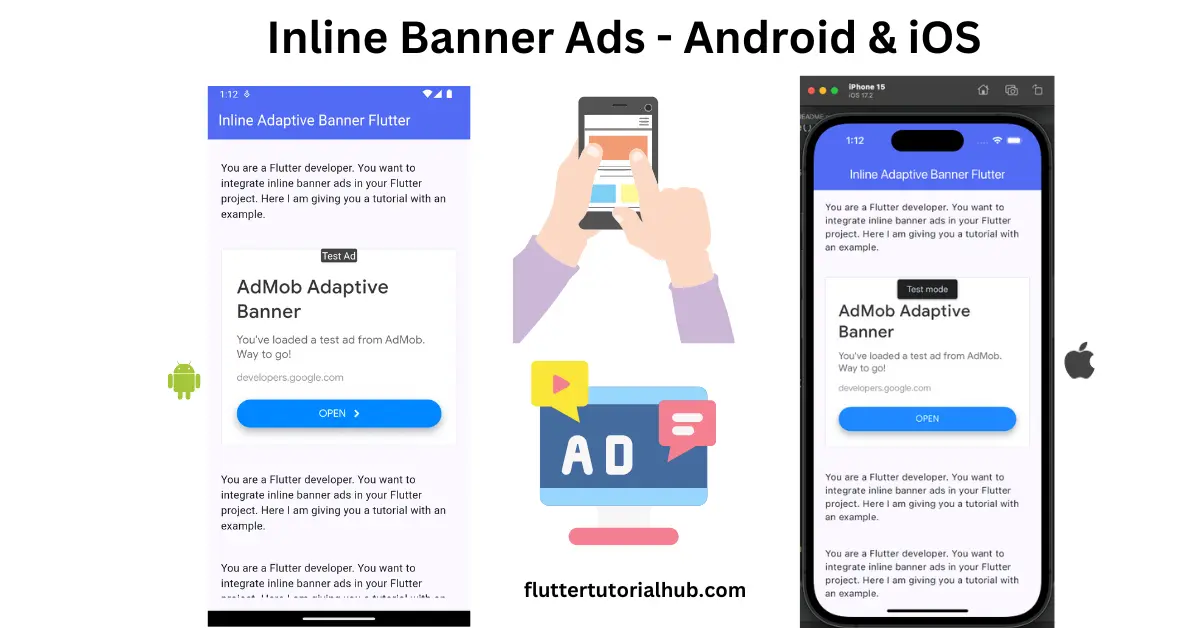
Inline Banner Ad Sizes
There are 3 types of banners that will automatically display as per your content.
- Small Banner: It is displayed as a standard size (320×50).
- Medium Rectangle: It is displayed some larger than standard size (300×250).
- Adaptive Banner: It will be automatically adjusted to fit the screen width.
Conclusion
Integrating inline adaptive banner ads into your Flutter app can help to monetize your content with a better user experience. This ad basically adjusts to fit the screen width with different devices responsive. You can easily implement it rather than native ads, interstitial ads, or rewarded video ads.
Frequently Asked Questions(FAQs)
How do I set banner ads in Flutter?
You can set banner ads in Flutter in two ways. You can implement inline banner ads and adaptive banner ads in your Flutter app. To implement it, you can follow the steps provided in this article.
How do I add banner ads to my app?
To add banner ads in your app, you need to create an admob account. You need to create your application under the account. You can create various add-on formats in your application. You have to create a banner ad ID from there. You can follow the steps to integrate ads in your app.
Are banner ads cheap?
Yes, banner ads are cheaper than native, rewarded video ads. The CPC (cost per click) is very few cents to few dollars. It depends on the geolocation and category of your app.
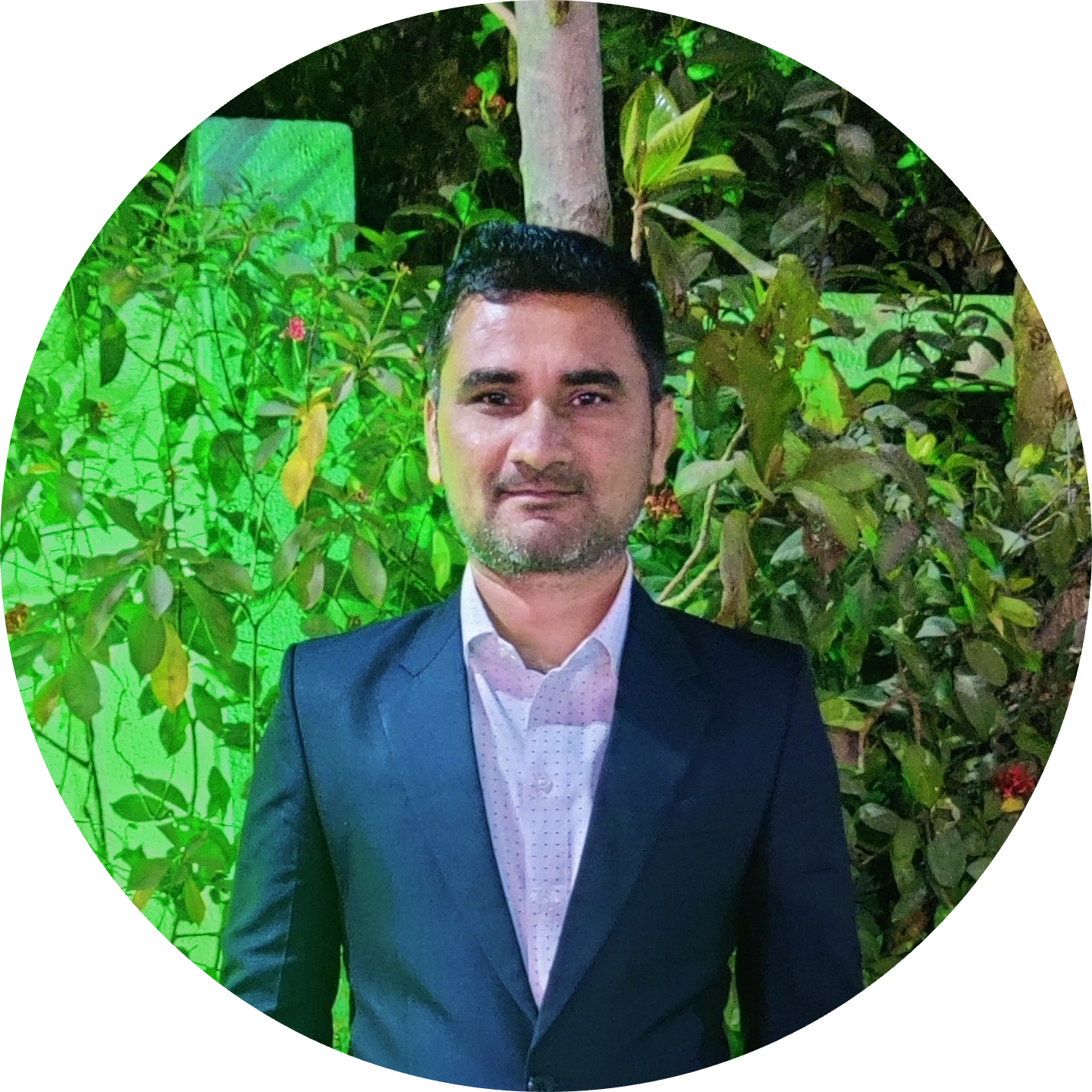
I’m a Flutter and iOS developer, entrepreneur, and owner of fluttertutorialhub.com. I live in India, and I love to write tutorials and tips that can help other developers. I am a big fan of Flutter, FlutterFlow, iOS, Android, mobile application development, and dart programming languages from the early stages. I believe in hard work and consistency.
Hire me on Upwork: https://www.upwork.com/freelancers/~01b4147994e5007ffc