Google AdMob Rewarded Video Ads is an ad format that allows users to watch video ads while engagement. It is a highly revenue-generated ad format among the native ads, collapsible banner ads, interstitial ads, or banner ads of AdMob Network. In this article, we will see how to integrate rewarded video ads into Flutter.
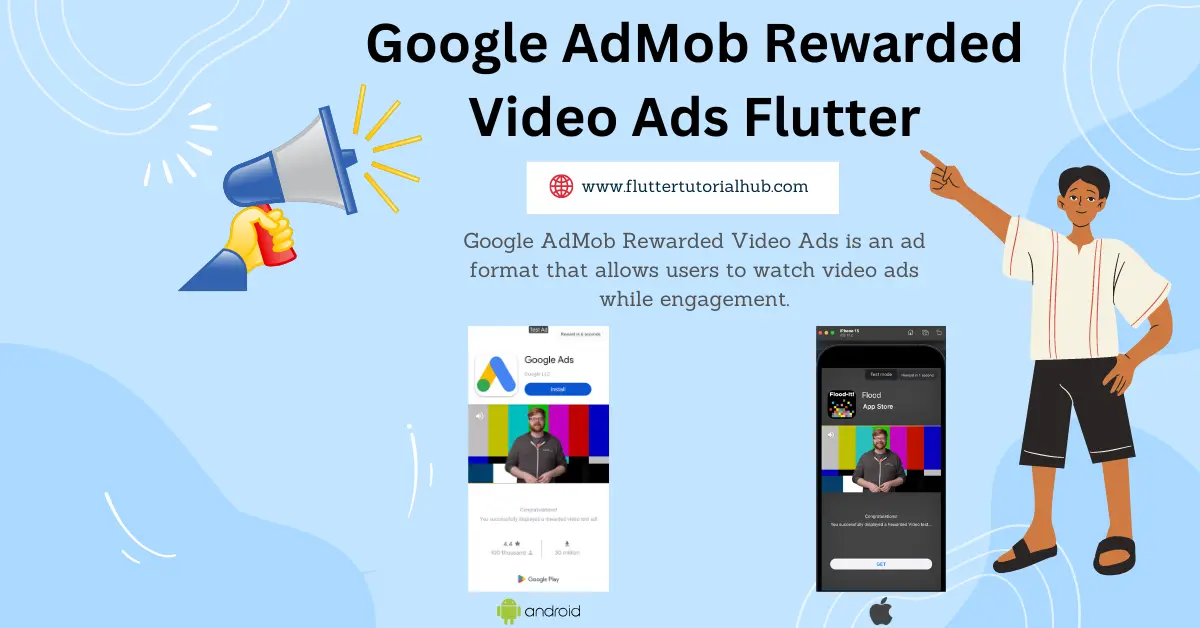
Table of Contents
Flutter admob rewarded ads allow users to earn some points or rewards or in-game items. It is displayed while the user finishes some levels or tasks in the application, in between the video ad display to gain some rewards for the user. It is a great way to earn more using this ad format.
How to Integrate Google AdMob Rewarded Ads in Flutter
We will see a step-by-step process to implement rewarded video ads in the Flutter project.
Step 1: Open the pubspec.yaml file in your Flutter project
- Add the google_mobile_ads package under the dependencies section. If you want to add a reward image to your project, then you need to add assets to your application. You can see code for the pubspec.yaml file below.
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.6
google_mobile_ads: ^5.1.0
# To add assets to your application, add an assets section, like this:
assets:
- images/
- Click on Pub get to retrieve packages in the project.
Step 2: Open the main.dart file in your Flutter project
- import header file for Google Mobile Ads.
import 'package:google_mobile_ads/google_mobile_ads.dart';
- Initialize the Mobile Ads SDK in main.dart file
void main() {
WidgetsFlutterBinding.ensureInitialized();
MobileAds.instance.initialize();
runApp(const MyApp());
}
- Initialize RewardedAd object. Also declare a variable named rewardPoints to display rewards for users.
RewardedAd? _rewardedAd;
var rewardPoints = 0;
- Declare add units for rewarded ads for Android and iOS. This is a test unit to display ads. For revenue generation, you have to create a new ad format called Rewarded Ads in your application of AdMob Account.
final adUnitId = Platform.isAndroid
? 'ca-app-pub-3940256099942544/5224354917'
: 'ca-app-pub-3940256099942544/1712485313';
- In the initState() method, you need to load rewarded video ads before using it.
@override
void initState() {
// TODO: implement initState
super.initState();
loadAd();
}
void loadAd() {
RewardedAd.load(
adUnitId: adUnitId,
request: const AdRequest(),
rewardedAdLoadCallback: RewardedAdLoadCallback(onAdLoaded: (ad) {
ad.fullScreenContentCallback = FullScreenContentCallback(
onAdShowedFullScreenContent: (ad) {},
onAdImpression: (ad) {},
onAdFailedToShowFullScreenContent: (ad, err) {
ad.dispose();
},
onAdDismissedFullScreenContent: (ad) {
ad.dispose();
},
onAdClicked: (ad) {});
_rewardedAd = ad;
}, onAdFailedToLoad: (LoadAdError error) {
print('RewardedAd failed to load: $error');
}));
}
- After successfully loading ads, you need to show ads by calling the showAds() method.
void showAds() {
_rewardedAd?.show(
onUserEarnedReward: (AdWithoutView ad, RewardItem rewardItem) {
// Reward the user for watching an ad.
print("Reward points:- ${rewardItem.amount}");
rewardPoints+= rewardItem.amount.toInt();
setState(() {
rewardPoints;
print("Reward:- $rewardPoints");
});
});
}
Step 3: Android Setup – Modify AndroidManifest.xml
- Add test AdMob AppId to your in AndroidManifestfile by adding the <meta-data> tag inside the
<application>
tag.
<manifest>
<application>
<meta-data
android:name="com.google.android.gms.ads.APPLICATION_ID"
android:value="ca-app-pub-3940256099942544~3347511713" />
</application>
</manifest>
Step 4: iOS Setup – Modify info.plist
- Add Admob App ID in the info.plist file.
<key>GADApplicationIdentifier</key>
<string>ca-app-pub-3940256099942544~1458002511</string>
Step 5: full code to display Google AdMob Rewarded Video Ads in the main.dart file.
import 'dart:io';
import 'package:flutter/material.dart';
import 'package:google_mobile_ads/google_mobile_ads.dart';
void main() {
WidgetsFlutterBinding.ensureInitialized();
MobileAds.instance.initialize();
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Google Admob Rewarded Ads',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.blue),
useMaterial3: true,
),
debugShowCheckedModeBanner: false,
home: const MyHomePage(title: 'Google Admob Rewarded Ads'),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
RewardedAd? _rewardedAd;
var rewardPoints = 0;
final adUnitId = Platform.isAndroid
? 'ca-app-pub-3940256099942544/5224354917'
: 'ca-app-pub-3940256099942544/1712485313';
@override
void initState() {
// TODO: implement initState
super.initState();
loadAd();
}
void loadAd() {
RewardedAd.load(
adUnitId: adUnitId,
request: const AdRequest(),
rewardedAdLoadCallback: RewardedAdLoadCallback(onAdLoaded: (ad) {
ad.fullScreenContentCallback = FullScreenContentCallback(
onAdShowedFullScreenContent: (ad) {},
onAdImpression: (ad) {},
onAdFailedToShowFullScreenContent: (ad, err) {
ad.dispose();
},
onAdDismissedFullScreenContent: (ad) {
ad.dispose();
},
onAdClicked: (ad) {});
_rewardedAd = ad;
}, onAdFailedToLoad: (LoadAdError error) {
print('RewardedAd failed to load: $error');
}));
}
void showAds() {
_rewardedAd?.show(
onUserEarnedReward: (AdWithoutView ad, RewardItem rewardItem) {
// Reward the user for watching an ad.
print("Reward points:- ${rewardItem.amount}");
rewardPoints+= rewardItem.amount.toInt();
setState(() {
rewardPoints;
print("Reward:- $rewardPoints");
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.blue,
foregroundColor: Colors.white,
title: Text(widget.title),
centerTitle: Platform.isAndroid ? false : true,
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
children: <Widget>[
const SizedBox(height: 100),
const Text(
'You will get 10 points for watching video ads.',
style: TextStyle(fontSize: 16, fontWeight: FontWeight.w600,color: Colors.black),
),
const SizedBox(height: 20),
SizedBox(
height: 100,
width: 100,
child: Image.asset(
"images/reward.png",
fit: BoxFit.fill,
),
),
const SizedBox(height: 20),
Text(
'Rewards Points:- $rewardPoints',
style: const TextStyle(fontSize: 20, fontWeight: FontWeight.w500),
),
const SizedBox(height: 50),
Container(
width: 100,
height: 50,
color: Colors.blue,
child: TextButton(
onPressed: () {
showAds();
},
child: const Text('Show Ads',
style: TextStyle(
fontSize: 14,
fontWeight: FontWeight.w500,
color: Colors.white))),
)
],
),
),
);
}
}
Step 6: Run the project!
Android Output:
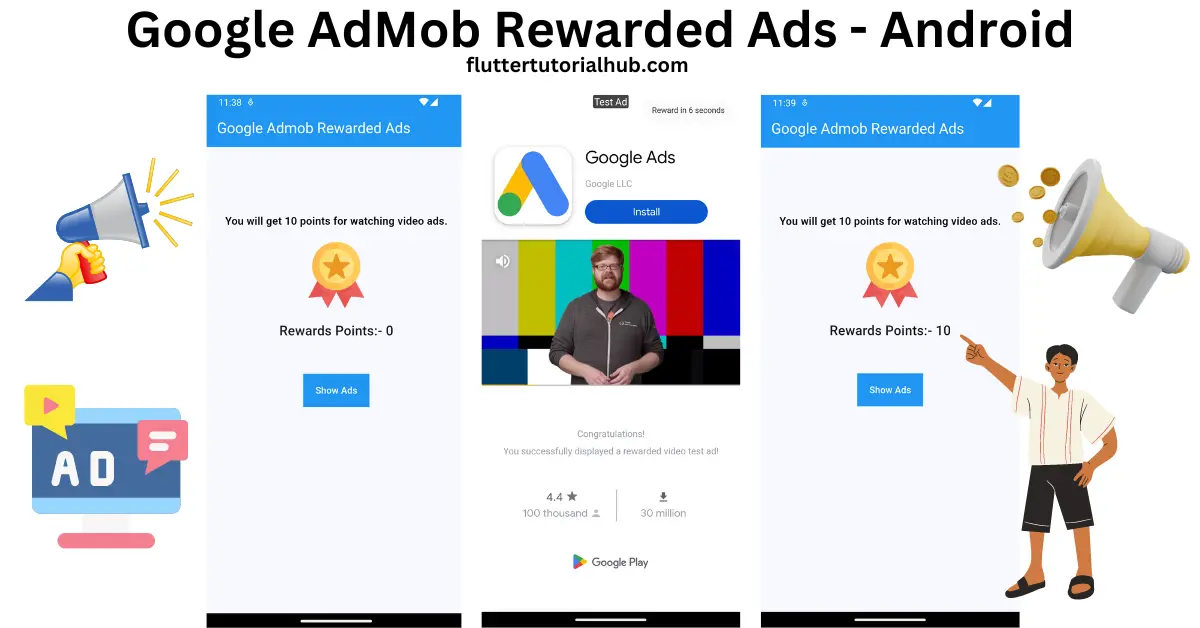
iOS Output: Run the project in the iPhone 15 simulator
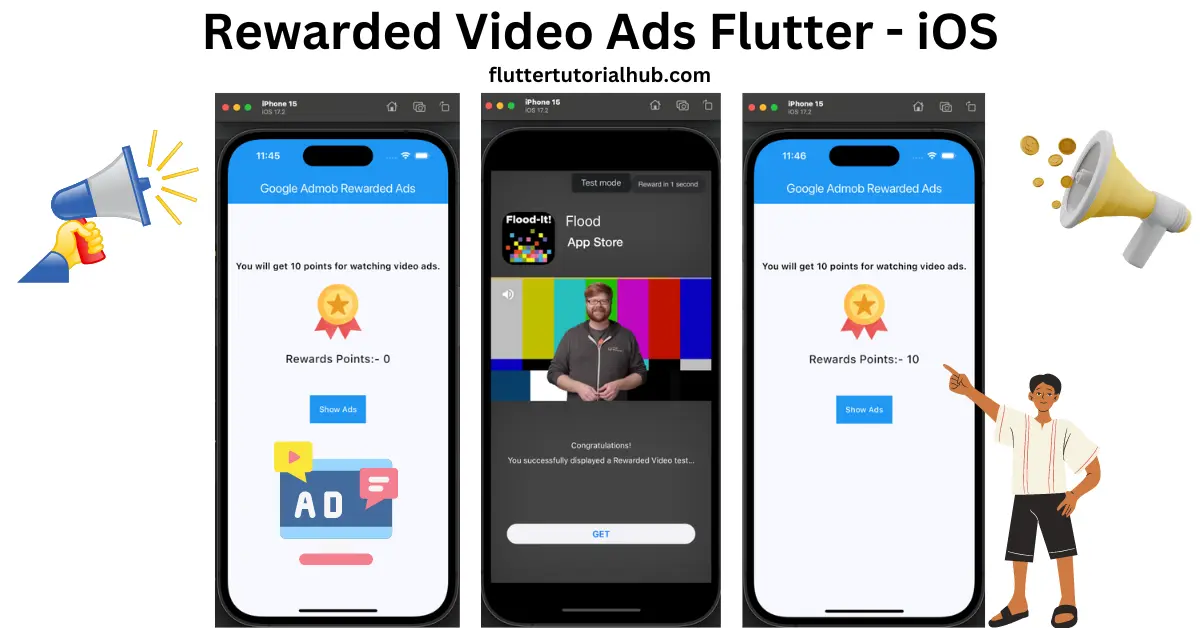
Conclusion
Implementing admob-rewarded ads in your Flutter project is a great way to increase user engagement. It allows win-win situations for users and publishers. It is very easy to integrate. Make sure to use test IDs while developing the project. Once you complete it, you need to create live ad units for rewards. Make sure to replace ad units and app ID before going to release on the Google Play Store and Apple App Store.
Frequently Asked Questions(FAQs)
What is rewarded video ads?
Rewarded video ads are a type of video ad format that offers users a reward in exchange for watching the ad in full screen. It is commonly used in mobile apps. You can also setup it in a business-level application. You can display it while the user needs to wait for some functionality to be done. Most games where users can earn game rewards like extra lives or coins by watching a video ad.
Why am I unable to open any reward ads in Flutter Google Mobile Ads?
This step will help you integrate AdMob-rewarded ads into your Flutter app.
How do I show rewarded ads in Flutter?
To show rewarded ads in your Flutter project, you need to integrate the google_mobile_ads package in pubspec.yaml. You can see the detailed code to integrate rewarded video ads in the Flutter project.
Are rewarded ads worth it?
It is worth it in some cases. On the other side, with in-app purchases or subscription-based applications, users may decide to see video ads for a reward. It seems to decrease in revenue.
Are rewarded ads skippable?
Yes, admob-rewarded ads are skippable. The user can skip the video ads or stop viewing ads.
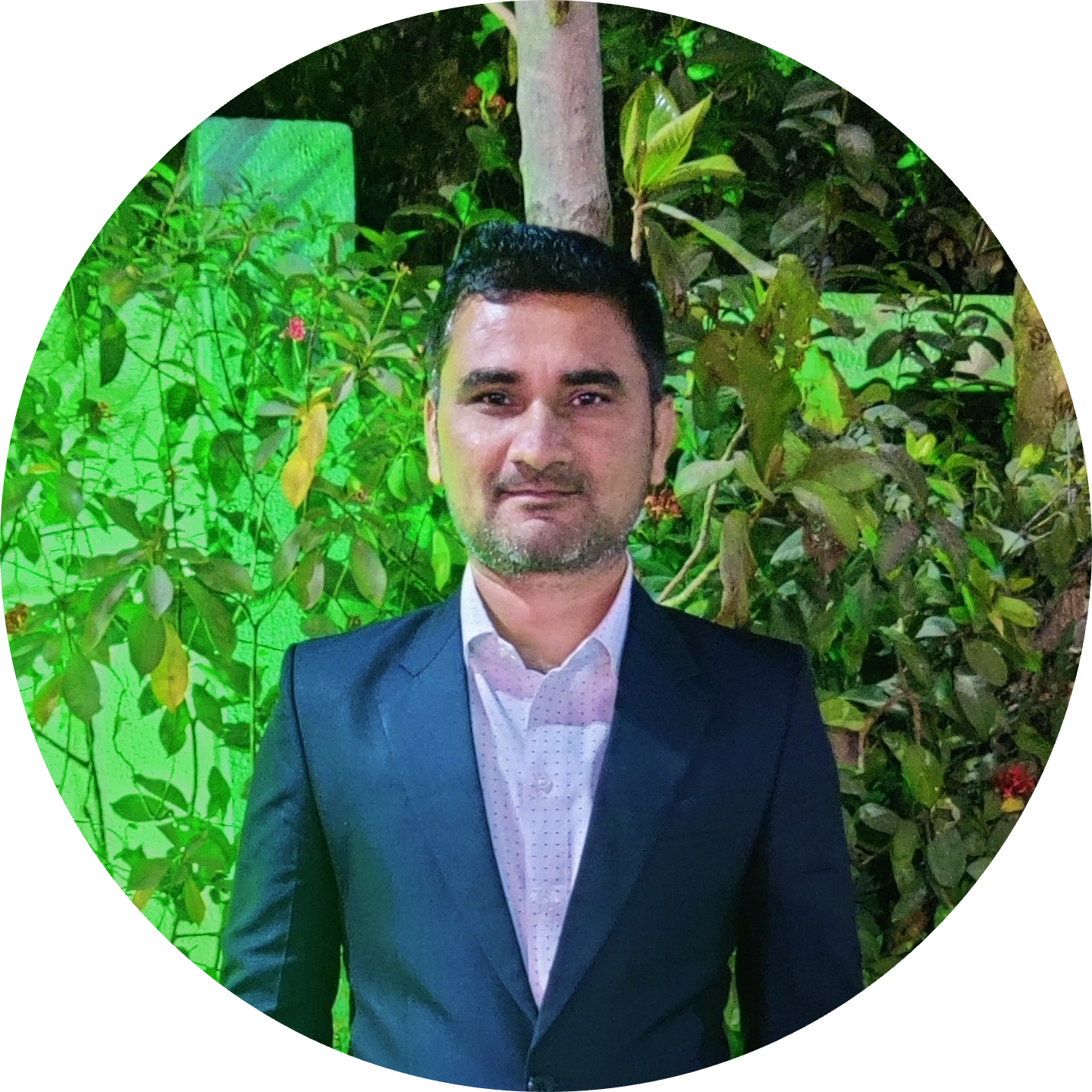
I’m a Flutter and iOS developer, entrepreneur, and owner of fluttertutorialhub.com. I live in India, and I love to write tutorials and tips that can help other developers. I am a big fan of Flutter, FlutterFlow, iOS, Android, mobile application development, and dart programming languages from the early stages. I believe in hard work and consistency.